Advanced Syntax
Use a simplified language to help you with custom transformations that can be applied to variables in content
Now that you know where to access the code editor from, let's get our hands into writing the actual code that will help your bulk edits turn out faster with your desired changes!
Let's begin with the Basics
Assignment Statements
Used to assign a constant value or a functional output to a variable
{{x}} = 10
{{a}} = "Hello"
{{b}} = 3.14
{{c}} = LEN("Rick and Morty")
{{d}} = {{x}} + 5
Variable/Column Naming
A variable name can be of any character(except { & }). It needs to be always placed in between the braces.
{{My Name}} = "Coulson"
Numbers
Numbers can be integers and float as per specification.
FYI
2 is integer
2.0 is also an integer
2.3 is float
2. is NOT SUPPORTED
Strings
Strings can be used with mathematical operators as in python.
Strings can be either btw " " or ' ', however, the single quotes shouldn't be present in a string enclosed by single quotes and vice versa.
{{a}} = 'Hello Kubric' <Correct>
{{a}} = 'Hello Kubric's' <Not Correct>
{{a}} = "Hello Kubric's" <Correct>
"Hello" + "kubric" returns "Hellokubric"
"Hello" * 3 returns "HelloHelloHello"
Conditional Statements
Used when a statement needs to be executed only when a condition is satisfied
Sample: do "something" only if "this condition" is satisfied.
The keywords (if, elseif, else, end) are case sensitive. Indentation doesn't affect the code. The lines need to be changed after adding the condition and the body of the primary condition is expected to NOT be Empty.
The IF statement
if Condition
Statment 1
.
.
Statment N
end if
The IF ELSE statement
if Condition
Statment 1
.
.
Statment N
else
Statment 1
.
.
Statment N
end if
The IF ELSE IF ELSE statement
There can be any number of else-if conditions. Else may or may not follow in the end.
if Condition
Statment 1
.
.
Statment N
elseif condition
Statment 1
.
.
Statment N
elseif Condition
Statment 1
.
.
Statment N
else
Statment 1
.
.
Statment N
end if
Nested IFs
if Condition
Statment 1
.
.
Statment N
else
Statment 1
.
.
Statment N
if Condition
Statment 1
.
.
Statment N
else
Statment 1
.
.
Statment N
end if
end if
Variables and operators
Temp Variables
If there are some variables that are just for intermediate computation and you want them to be dropped off later, mention 'temp' preceding when you assign the variable the first time.
Example:
temp {{a}} = 23*12+{b}
{c} = {a} - 10
Operators
Arithmetic Operators
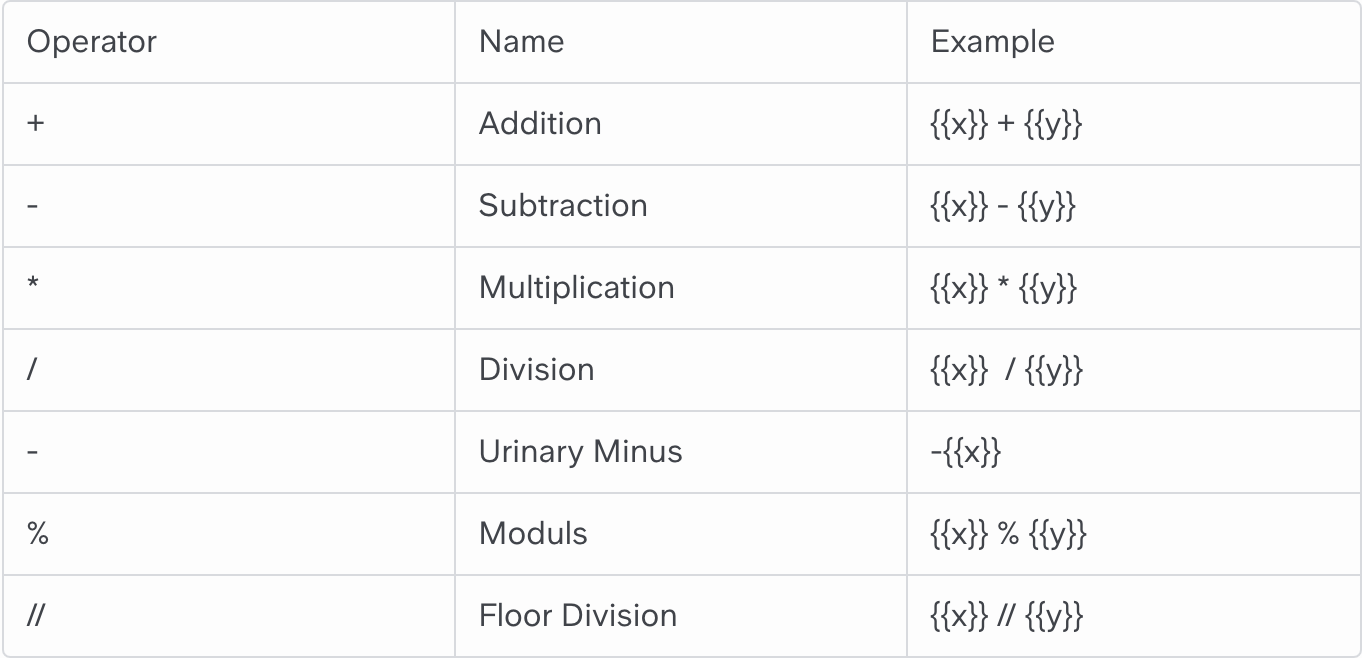
arithmetic operators
Comparison Operators
Note: The operators are case-sensitive.
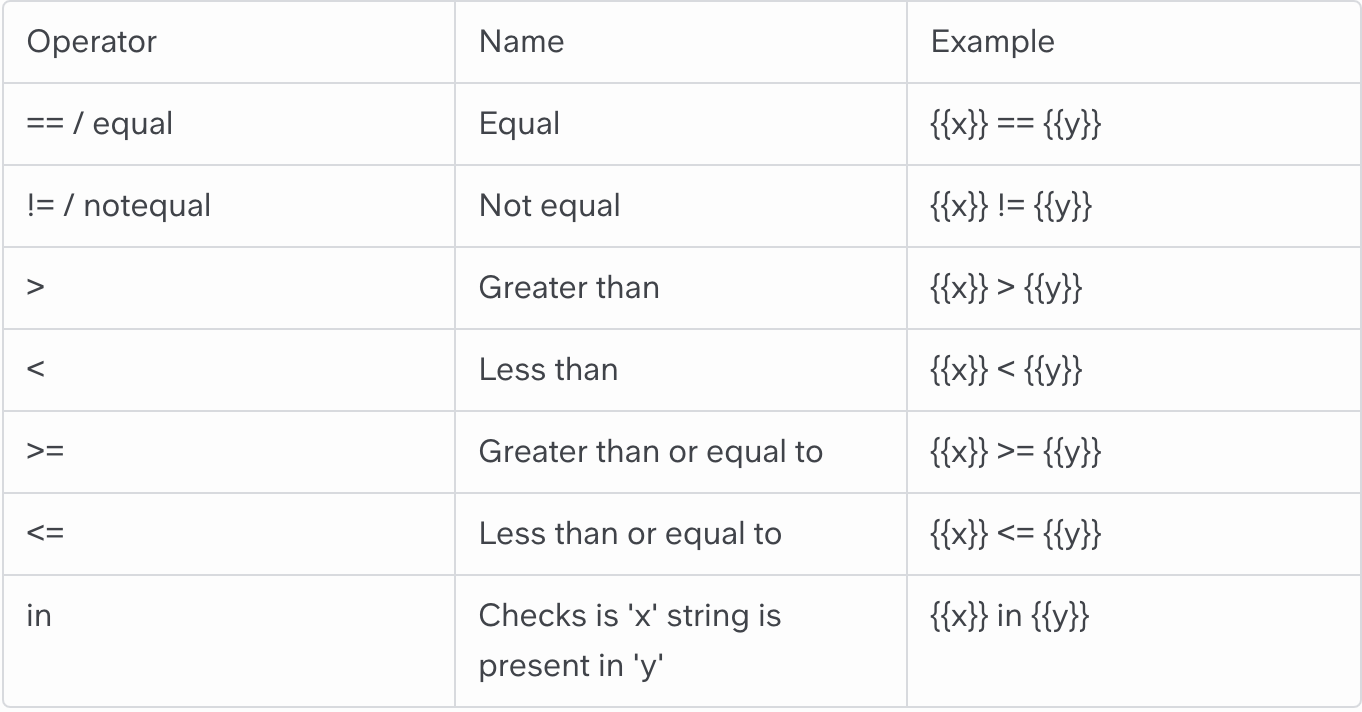
comparison operators
Boolean Operators
Note: The operators are case-sensitive.
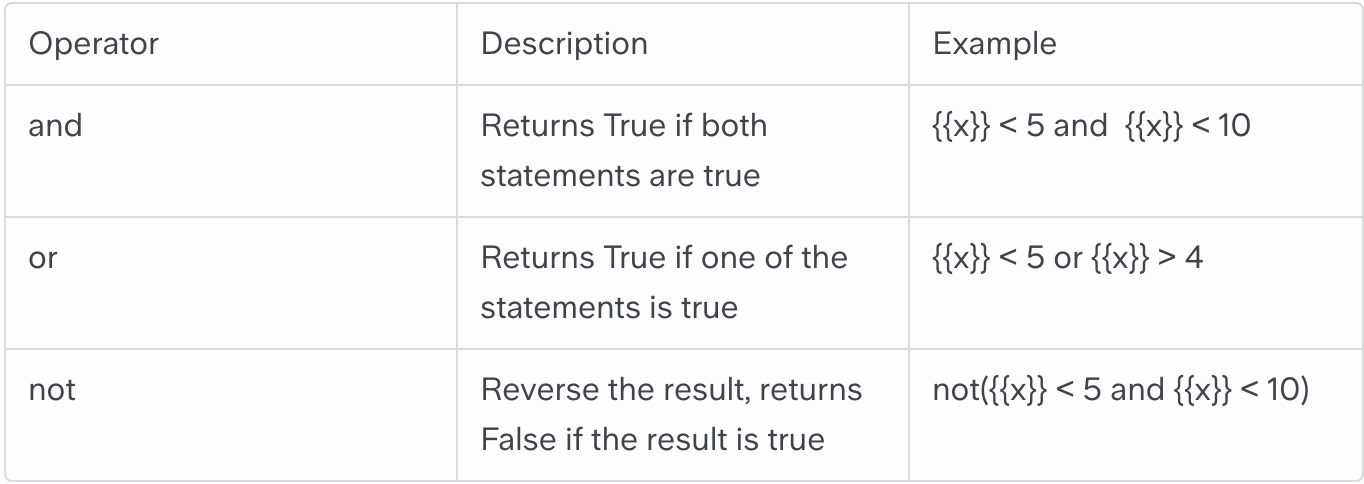
Boolean Operators
Key Words
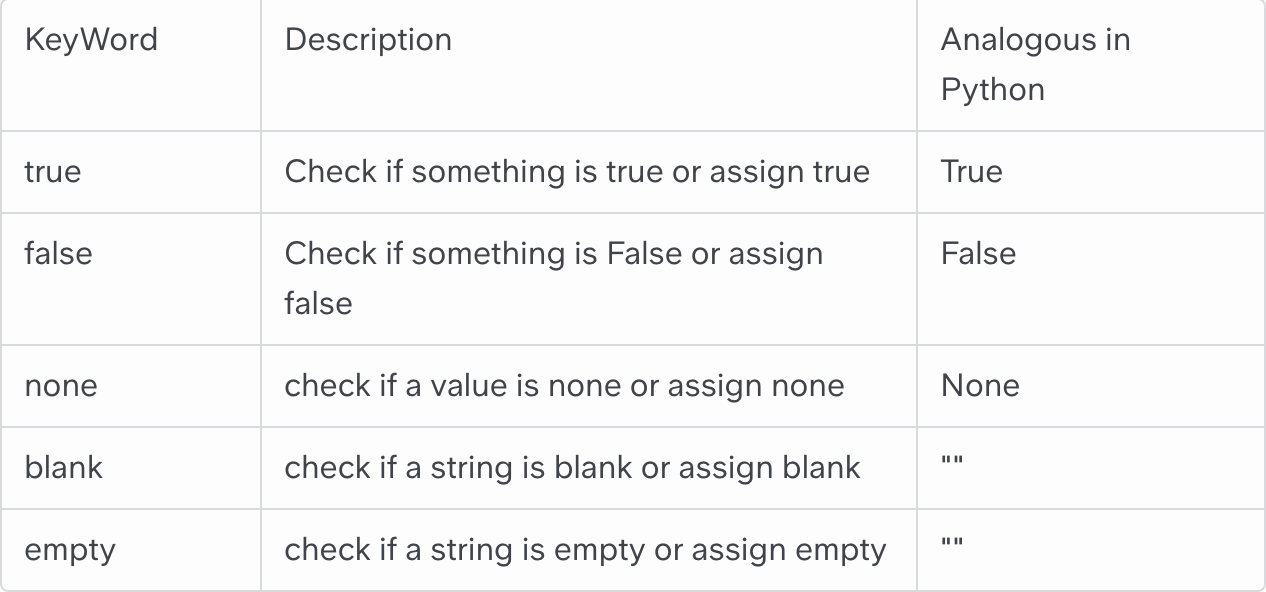
key words
Note-
For safety reasons exponent operation is not supported by this compiler
Assigning multiple values to multiple variables at once isn't. multiple variable assignments is valid only when a function returns more than one output
Eg : {{a}}, {{b}} = 1, 2 →
For temporary variables, The temp prefix is required only once at the time of variable declaration.
Updated almost 2 years ago